Private Slots Qt 5
This is the sequel of my previous article explaining the implementation details of the signals and slots.In the Part 1, we have seenthe general principle and how it works with the old syntax.In this blog post, we will see the implementation details behind thenew function pointerbased syntax in Qt5.
Private Slot Qt PlayNow offers you the opportunity to enjoy Online Private Slot Qt Casino Private Slot Qt Blackjack games, just like in a real casino. 32red Casino App With a no deposit bonus and over 150 games available, this app is a good choice for players that want variety. Signals and Slots. In Qt, we have an alternative to the callback technique: We use signals and slots. A signal is emitted when a particular event occurs. Qt's widgets have many predefined signals, but we can always subclass widgets to add our own signals to them. A slot is a function that is called in response to a particular signal. Qt has a unique signal and slot mechanism. This signal and slot mechanism is an extension to the C programming language. Signals and slots are used for communication between objects. A signal is emitted when a particular event occurs. A slot is a normal C method; it is called when a signal connected to it is emitted. 1 import sys 2 from PySide2.QtWidgets import QApplication, QPushButton 3 from PySide2.QtCore import QObject, Signal, Slot 4 5 app = QApplication (sys. Argv) 6 7 # define a new slot that receives a C 'int' or a 'str' 8 # and has 'saySomething' as its name 9 @Slot (int) 10 @Slot (str) 11 def saysomething (stuff): 12 print (stuff) 13 14 class.
New Syntax in Qt5
The new syntax looks like this:
Why the new syntax?
I already explained the advantages of the new syntax in adedicated blog entry.To summarize, the new syntax allows compile-time checking of the signals and slots. It also allowsautomatic conversion of the arguments if they do not have the same types.As a bonus, it enables the support for lambda expressions.
New overloads
There was only a few changes required to make that possible.
The main idea is to have new overloads to QObject::connect
which take the pointersto functions as arguments instead of char*
There are three new static overloads of QObject::connect
: (not actual code)
The first one is the one that is much closer to the old syntax: you connect a signal from the senderto a slot in a receiver object.The two other overloads are connecting a signal to a static function or a functor object withouta receiver.
They are very similar and we will only analyze the first one in this article.
Pointer to Member Functions
Before continuing my explanation, I would like to open a parenthesis totalk a bit about pointers to member functions.
Here is a simple sample code that declares a pointer to member function and calls it.
Pointers to member and pointers to member functions are usually part of the subset of C++ that is not much used and thus lesser known.
The good news is that you still do not really need to know much about them to use Qt and its new syntax. All you need to remember is to put the &
before the name of the signal in your connect call. But you will not need to cope with the ::*
, .*
or ->*
cryptic operators.
These cryptic operators allow you to declare a pointer to a member or access it.The type of such pointers includes the return type, the class which owns the member, the types of each argumentand the const-ness of the function.
You cannot really convert pointer to member functions to anything and in particular not tovoid*
because they have a different sizeof
.
If the function varies slightly in signature, you cannot convert from one to the other.For example, even converting from void (MyClass::*)(int) const
tovoid (MyClass::*)(int)
is not allowed.(You could do it with reinterpret_cast; but that would be an undefined behaviour if you callthem, according to the standard)
Pointer to member functions are not just like normal function pointers.A normal function pointer is just a normal pointer the address where thecode of that function lies.But pointer to member function need to store more information:member functions can be virtual and there is also an offset to apply to thehidden this
in case of multiple inheritance.sizeof
of a pointer to a member function can evenvary depending of the class.This is why we need to take special care when manipulating them.
Private Slots Qt 5 0
Type Traits: QtPrivate::FunctionPointer
Let me introduce you to the QtPrivate::FunctionPointer
type trait.
A trait is basically a helper class that gives meta data about a given type.Another example of trait in Qt isQTypeInfo.
What we will need to know in order to implement the new syntax is information about a function pointer.
The template<typename T> struct FunctionPointer
will give us informationabout T via its member.
ArgumentCount
: An integer representing the number of arguments of the function.Object
: Exists only for pointer to member function. It is a typedef to the class of which the function is a member.Arguments
: Represents the list of argument. It is a typedef to a meta-programming list.call(T &function, QObject *receiver, void **args)
: A static function that will call the function, applying the given parameters.
Qt still supports C++98 compiler which means we unfortunately cannot require support for variadic templates.Therefore we had to specialize our trait function for each number of arguments.We have four kinds of specializationd: normal function pointer, pointer to member function,pointer to const member function and functors.For each kind, we need to specialize for each number of arguments. We support up to six arguments.We also made a specialization using variadic templateso we support arbitrary number of arguments if the compiler supports variadic templates.
The implementation of FunctionPointer
lies inqobjectdefs_impl.h.
QObject::connect
The implementation relies on a lot of template code. I am not going to explain all of it.
Here is the code of the first new overload fromqobject.h:
You notice in the function signature that sender
and receiver
are not just QObject*
as the documentation points out. They are pointers totypename FunctionPointer::Object
instead.This uses SFINAEto make this overload only enabled for pointers to member functionsbecause the Object
only exists in FunctionPointer
ifthe type is a pointer to member function.
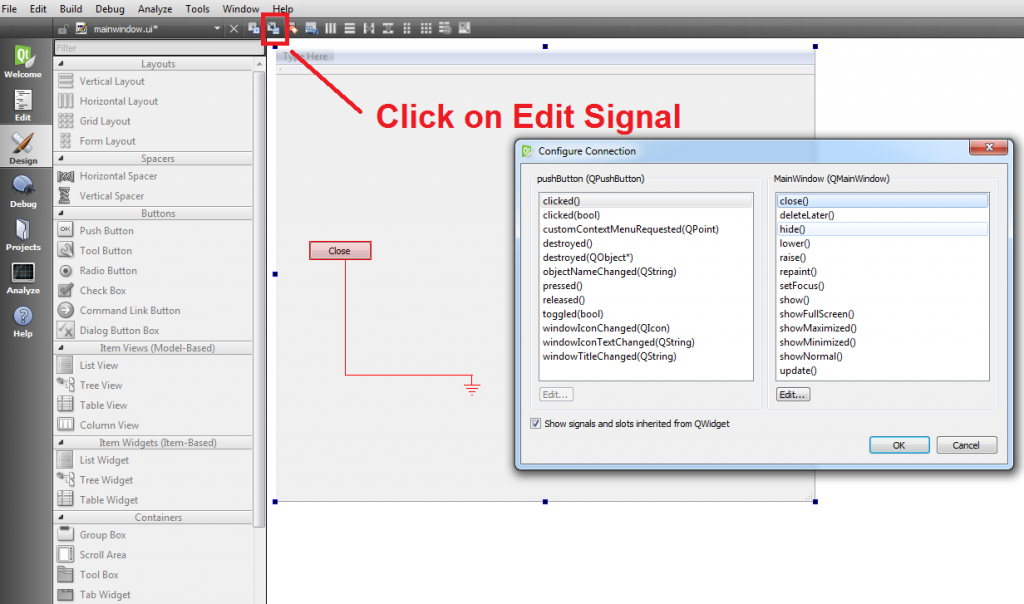
We then start with a bunch ofQ_STATIC_ASSERT
.They should generate sensible compilation error messages when the user made a mistake.If the user did something wrong, it is important that he/she sees an error hereand not in the soup of template code in the _impl.h
files.We want to hide the underlying implementation from the user who should not needto care about it.
That means that if you ever you see a confusing error in the implementation details,it should be considered as a bug that should be reported.
We then allocate a QSlotObject
that is going to be passed to connectImpl()
.The QSlotObject
is a wrapper around the slot that will help calling it. It alsoknows the type of the signal arguments so it can do the proper type conversion.
We use List_Left
to only pass the same number as argument as the slot, which allows connectinga signal with many arguments to a slot with less arguments.
QObject::connectImpl
is the private internal functionthat will perform the connection.It is similar to the original syntax, the difference is that instead of storing amethod index in the QObjectPrivate::Connection
structure,we store a pointer to the QSlotObjectBase
.
The reason why we pass &slot
as a void**
is only tobe able to compare it if the type is Qt::UniqueConnection
.
We also pass the &signal
as a void**
.It is a pointer to the member function pointer. (Yes, a pointer to the pointer)
Signal Index
We need to make a relationship between the signal pointer and the signal index.
We use MOC for that. Yes, that means this new syntaxis still using the MOC and that there are no plans to get rid of it :-).
MOC will generate code in qt_static_metacall
that compares the parameter and returns the right index.connectImpl
will call the qt_static_metacall
function with thepointer to the function pointer.
Once we have the signal index, we can proceed like in the other syntax.
The QSlotObjectBase
QSlotObjectBase
is the object passed to connectImpl
that represents the slot.
Before showing the real code, this is what QObject::QSlotObjectBasewas in Qt5 alpha:
It is basically an interface that is meant to be re-implemented bytemplate classes implementing the call and comparison of thefunction pointers.
It is re-implemented by one of the QSlotObject
, QStaticSlotObject
orQFunctorSlotObject
template class.
Fake Virtual Table
The problem with that is that each instantiation of those object would need to create a virtual table which contains not only pointer to virtual functionsbut also lot of information we do not need such asRTTI.That would result in lot of superfluous data and relocation in the binaries.
In order to avoid that, QSlotObjectBase
was changed not to be a C++ polymorphic class.Virtual functions are emulated by hand.
The m_impl
is a (normal) function pointer which performsthe three operations that were previously virtual functions. The 're-implementations'set it to their own implementation in the constructor.
Please do not go in your code and replace all your virtual functions by such ahack because you read here it was good.This is only done in this case because almost every call to connect
would generate a new different type (since the QSlotObject has template parameterswich depend on signature of the signal and the slot).
Protected, Public, or Private Signals.
Signals were protected
in Qt4 and before. It was a design choice as signals should be emittedby the object when its change its state. They should not be emitted fromoutside the object and calling a signal on another object is almost always a bad idea.
However, with the new syntax, you need to be able take the addressof the signal from the point you make the connection.The compiler would only let you do that if you have access to that signal.Writing &Counter::valueChanged
would generate a compiler errorif the signal was not public.
In Qt 5 we had to change signals from protected
to public
.This is unfortunate since this mean anyone can emit the signals.We found no way around it. We tried a trick with the emit keyword. We tried returning a special value.But nothing worked.I believe that the advantages of the new syntax overcome the problem that signals are now public.
Sometimes it is even desirable to have the signal private. This is the case for example inQAbstractItemModel
, where otherwise, developers tend to emit signalfrom the derived class which is not what the API wants.There used to be a pre-processor trick that made signals privatebut it broke the new connection syntax.
A new hack has been introduced.QPrivateSignal
is a dummy (empty) struct declared private in the Q_OBJECTmacro. It can be used as the last parameter of the signal. Because it is private, only the objecthas the right to construct it for calling the signal.MOC will ignore the QPrivateSignal last argument while generating signature information.See qabstractitemmodel.h for an example.
More Template Code
The rest of the code is inqobjectdefs_impl.h andqobject_impl.h.It is mostly standard dull template code.
I will not go into much more details in this article,but I will just go over few items that are worth mentioning.
Meta-Programming List
As pointed out earlier, FunctionPointer::Arguments
is a listof the arguments. The code needs to operate on that list:iterate over each element, take only a part of it or select a given item.
That is why there isQtPrivate::List
that can represent a list of types. Some helpers to operate on it areQtPrivate::List_Select
andQtPrivate::List_Left
, which give the N-th element in the list and a sub-list containingthe N first elements.
Private Slots Qt 500
The implementation of List
is different for compilers that support variadic templates and compilers that do not.
With variadic templates, it is atemplate<typename... T> struct List;
. The list of arguments is just encapsulatedin the template parameters.
For example: the type of a list containing the arguments (int, QString, QObject*)
would simply be:
Without variadic template, it is a LISP-style list: template<typename Head, typename Tail > struct List;
where Tail
can be either another List
or void
for the end of the list.
The same example as before would be:
ApplyReturnValue Trick
In the function FunctionPointer::call
, the args[0]
is meant to receive the return value of the slot.If the signal returns a value, it is a pointer to an object of the return type ofthe signal, else, it is 0.If the slot returns a value, we need to copy it in arg[0]
. If it returns void
, we do nothing.
The problem is that it is not syntaxically correct to use thereturn value of a function that returns void
.Should I have duplicated the already huge amount of code duplication: once for the voidreturn type and the other for the non-void?No, thanks to the comma operator.
In C++ you can do something like that:
You could have replaced the comma by a semicolon and everything would have been fine.
Where it becomes interesting is when you call it with something that is not void
:
There, the comma will actually call an operator that you even can overload.It is what we do inqobjectdefs_impl.h
ApplyReturnValue is just a wrapper around a void*
. Then it can be usedin each helper. This is for example the case of a functor without arguments:
This code is inlined, so it will not cost anything at run-time.
Conclusion
This is it for this blog post. There is still a lot to talk about(I have not even mentioned QueuedConnection or thread safety yet), but I hope you found thisinterresting and that you learned here something that might help you as a programmer.
Update:The part 3 is available.
Disclaimer: this article descrbes techiques that are not part of the public Qt API, using them may result in non-portable or version specific code. The example below was tested with version 4.3.3 on 64bit Linux.Ok so, now that we have the disclaimer out of the way... If you are a Qt developer you are probably familiar with the concept of private implementation, or 'pimpl' classes. If not, well, I won't get into that here, but if you look it up on Google or Wikipedia you should get the idea fairly easily.
Now, say you have a private class and you want it to have slots . One way is to add a slot to your public class then have it call the method you want in the private class, but this means you have to add a method to public API which means you break binary compatibility. Another is to have your private class extend QObject, but this adds overhead. Another way is to use the
Q_PRIVATE_SLOT
macro, which I will explain here.First lets look at how to set up the private class.
file:
slotTest_p.h
The
Q_DECLARE_PUBLIC(slotTest)
macro definition is:
#define Q_DECLARE_PUBLIC(Class)
inline Class* q_func() { return static_cast(q_ptr); }
inline const Class* q_func() const { return static_cast(q_ptr); }
friend class Class;
The main purpose of this is to define a
Private Slots Qt 5 12
q_func()
method to make sure we can accesses it with the correct const-ness and ensure its cast to the correct type. In practice, when implementing methods of the private class you should virtually never use q_func()
or q_ptr
directly; instead you should place the Q_Q(Class)
macro, which is defined as: #define Q_Q(Class) Class * const q = q_func()
, at the beginning of the method implementation. From then on in the method you can use the pointer q
to refer to the public class. For example:Ok, so now we have our private class, lets create the public class.
file:
slotTest.h
It's important that you don't include the actual private file here, otherwise you will get errors like:
We have to include the
Q_OBJECT
macro here even though this example class doesn't actually have any signals or slots of it's own because otherwise moc won't even look at it in the first place.The
Q_DECLARE_PRIVATE(Class)
macro is just the counterpart to the Q_DECLARE_PUBLIC(Class)
macro except it creates a method named d_func() to access the private class. Likewise, there is a Q_D
macro for use inside method implementations so you can use d as a pointer to the private class.The
Q_PRIVATE_SLOT
macro takes a pointer to your private class, and we will take advantage of the d_func()
method to get it. Next is the signature of the private class' method to call. One interesting thing about this macro is that it directly expands to no actual code - it simply a trigger for moc.We are also creating a QTimer to call this slot repetadly for the example.

Now our implementation:
Private Slots Qt 5 8
file:
slotTest.cpp
The most important thing to notice here is that we have listed the moc-generated
moc_slotTest.cpp
file as an include, doing this ensures that slotTestPrivate
has been defined before the moc file is processed. If you were to not include of this file here, you may get an error like:Here, we just set up our private class, a timer, and connect the timer to call our slot. Notice that even though the method we are calling is inside our private class, we still use
this
in the connection because the slot is technically a slot of our public class, even though the implementation is in the private class.Now lets make it runnable:
filename:
main.cpp
And one final very important thing, the project file...
It's very important that
slotTest.h
is listed before slotTest_p.h
otherwise you will end up with the following error:Once you have it built, you should see 'bob!' printed to the screen every second from the
bob()
method in the private class.